Formational Flocking C++ Demo
C++ Object Oriented.
1 week development time.
Personal project.
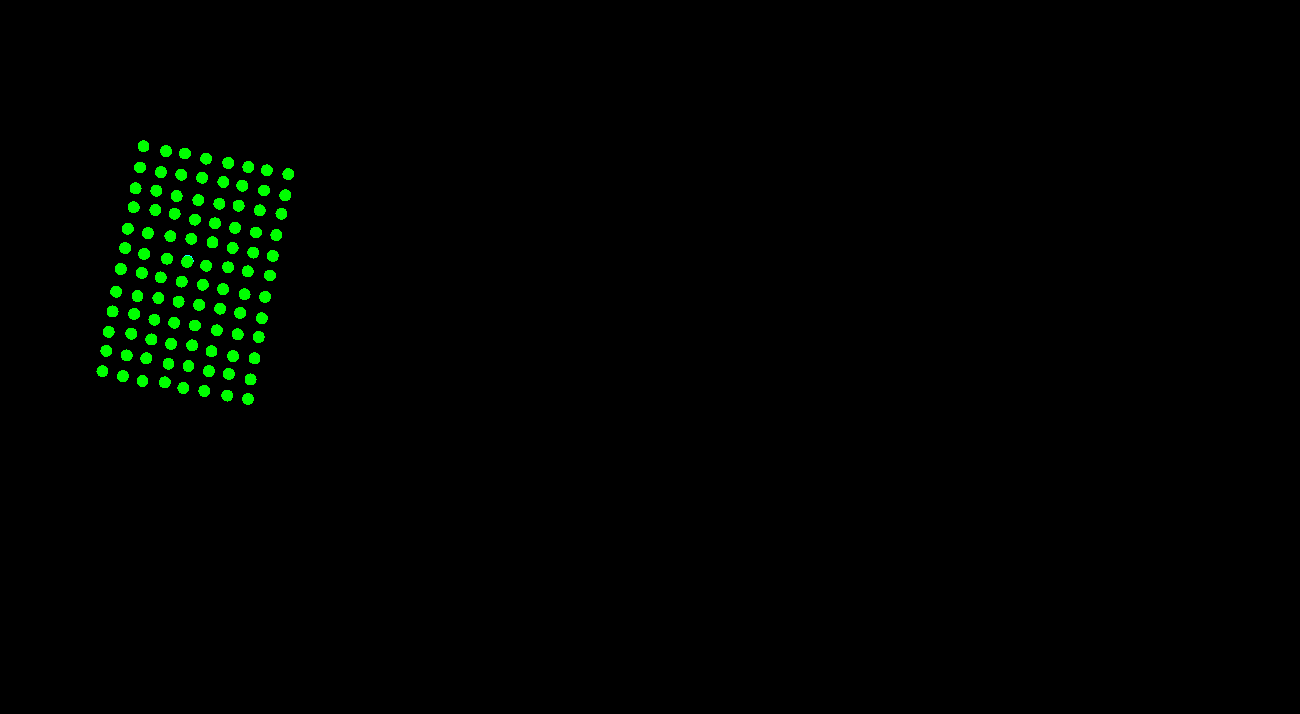
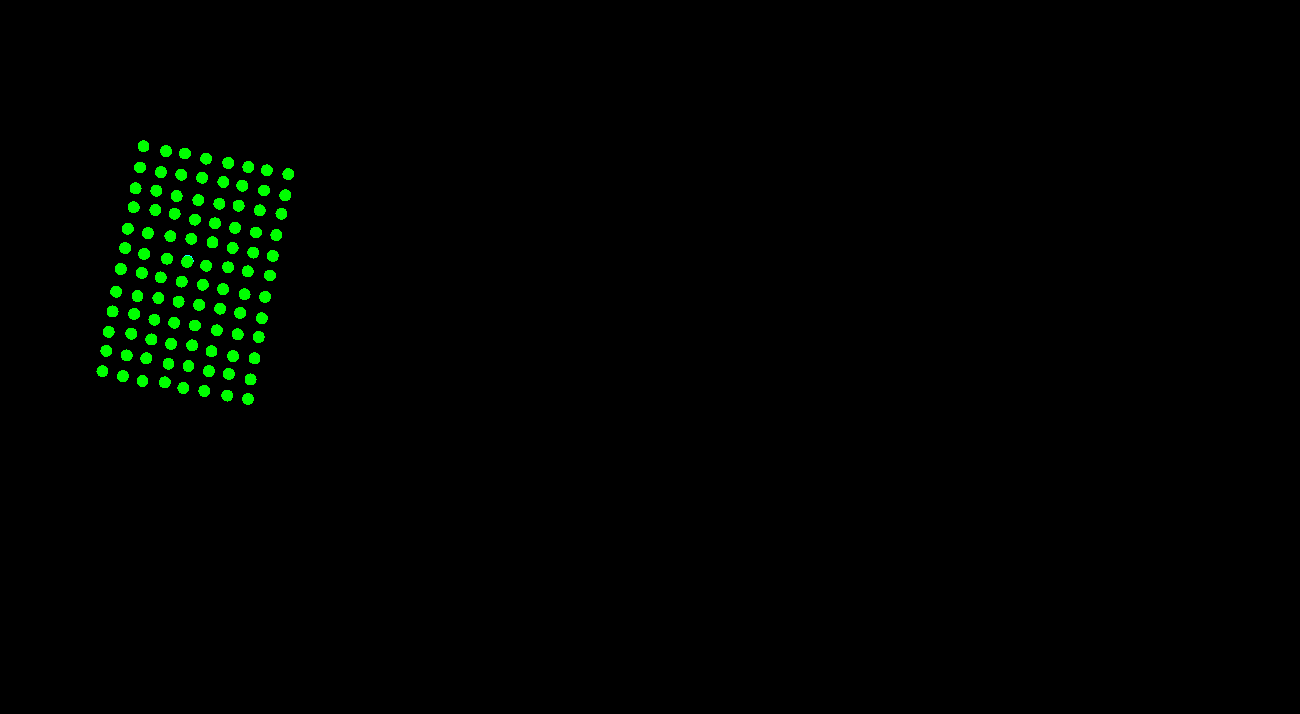
This project is a C++ Object-Oriented alternative to the Flocking Algorithm I developed for Védelem: The Golden Horde. This project was mainly meant for me to refresh and further improve my C++ skills.
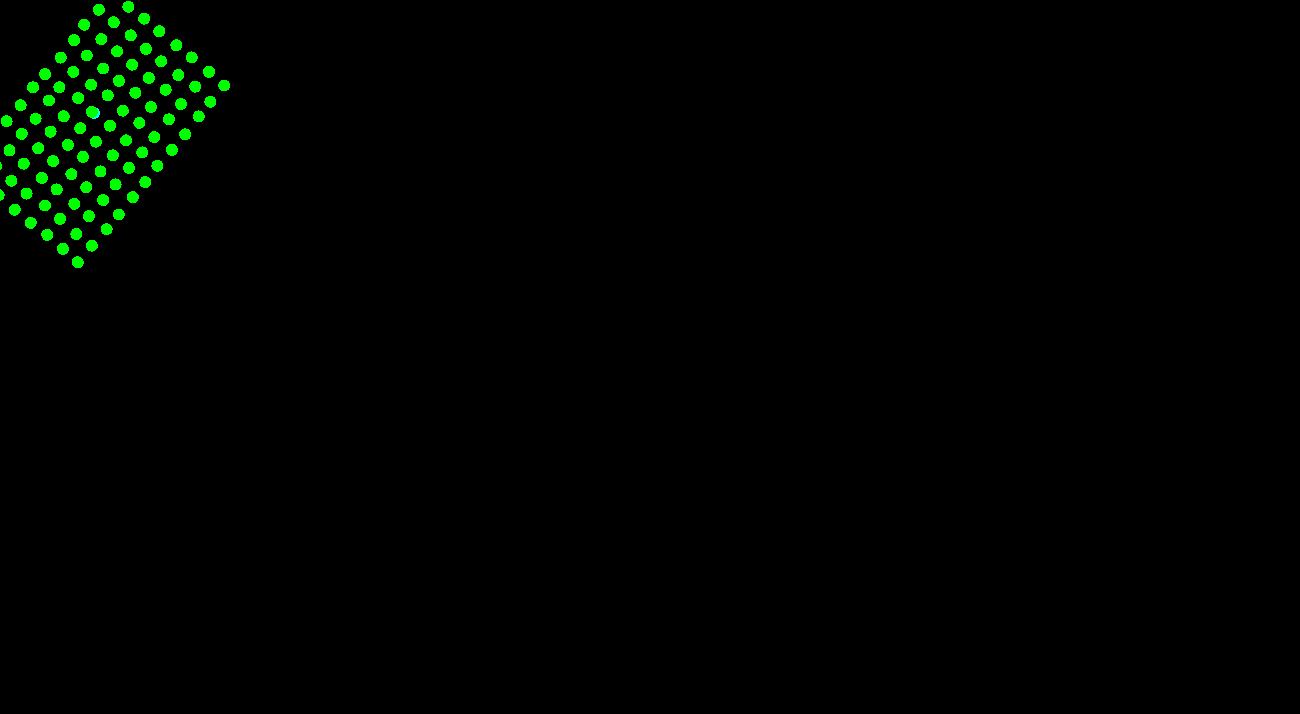
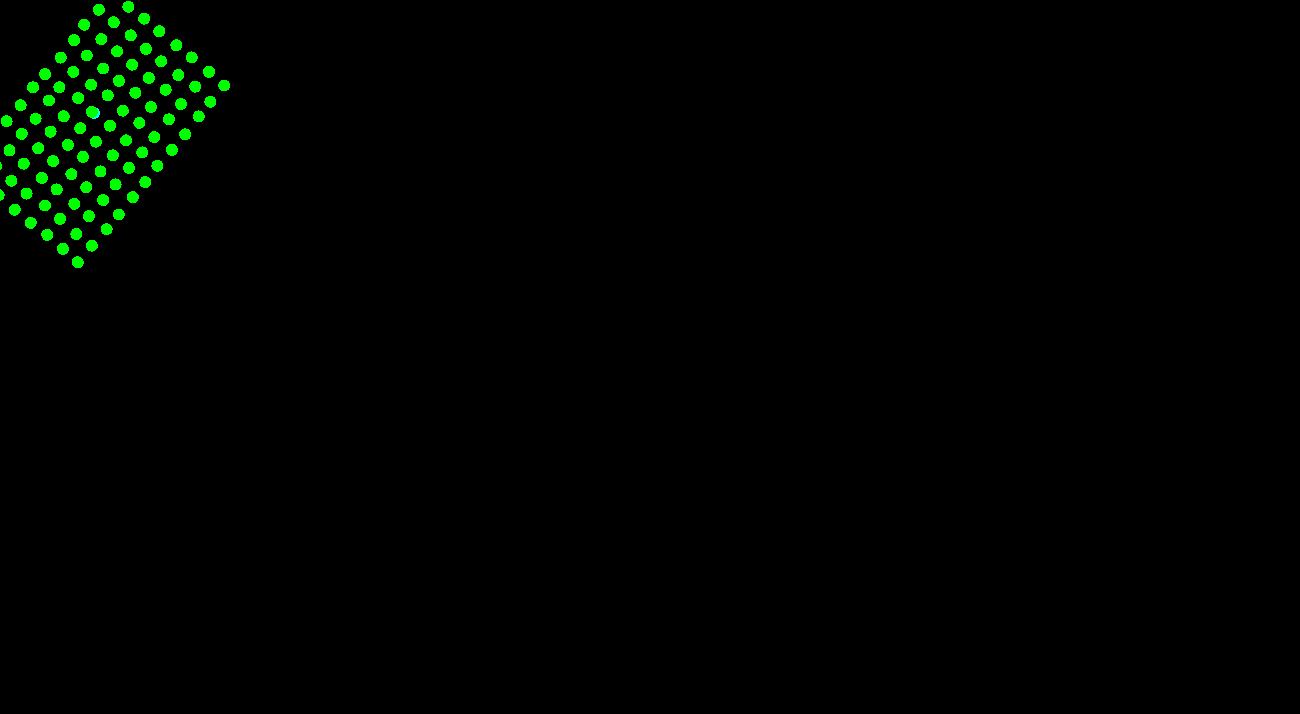
What is Formational Flocking Demo
My responsibilities:
This is a personal project. As such, I have written everything except for the 3 external libraries I am using.
Current functionality implemented:
- Rendering of units as green circles, and formation as blue circles.
- Importing of JSON values as variables used in the flocking algorithm at runtime.
- Spawning and Destroying formations.
- In the code specify the number of formations and their amount of units on the X-axis and Y-axis and the offset in-between units.
- Add and Remove units from a formation.
- Formation moves towards the cursor when the left mouse button is pressed.
- Units use the formational flocking algorithm to move with the formation object.
- Destroy a unit by pressing Del.
Planned functionality:
- Inputs to spawn and destroy formations.
- Selecting formations and moving only selected formations.
- Védelem inspired melee combat.
- Fixing some of the algorithm's know issues.
- When making a big turn the units overlap a lot.
- Units at the front get slowed down too much by the cohesion smoothing.
External Libraries:
SFML(Rendering), GLM(Math), Nlohmann/JSON(JSON Importer).
Detailed process:
The flocking algorithm is based on the algorithm that I wrote for all the unit behavior in Védelem: The Golden Horde but then rewritten into an Object-Oriented form in C++.
The algorithm itself is a modified version of the typical Cohesion, Alignment, Separation, but then Cohesion is overridden by the desired position in the formation. And uses field of view checks. As such there are no colliders used by this system.
The algorithm itself is optimized using a combined approach:
Step 1: Current implementation only checks for units in the same formation, but it is possible with a small change in the code to include specific other formations in the check.
Step 2: I do a cell calculation where every update I calculate a grid using the unit positions and the cellSize variable.
Step 3: The units check the distance between each other, and if they are within a specific distance, they use an angle calculation to check if the other unit is within the field of view.
Step 4: Only then the actual flocking calculations get run until it hits the maxPerceived variable set in the JSON.
In order to see the result of the algorithm, both as visual evidence and as debug tool I am using SFML for the rendering.
I also implemented Nlohmann his JSON importer into the project so I didn't have to hardcode any variables in, but instead now all the values used by the flocking algorithm are loaded in from a JSON file that looks like the picture on the right.
Code:
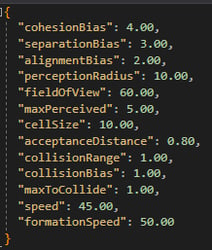
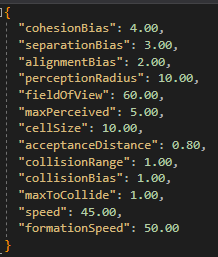
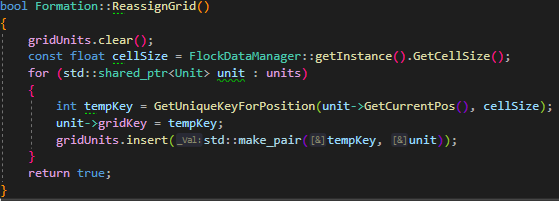
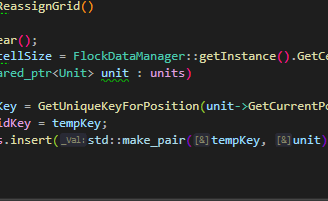

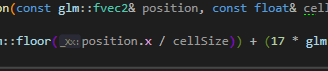
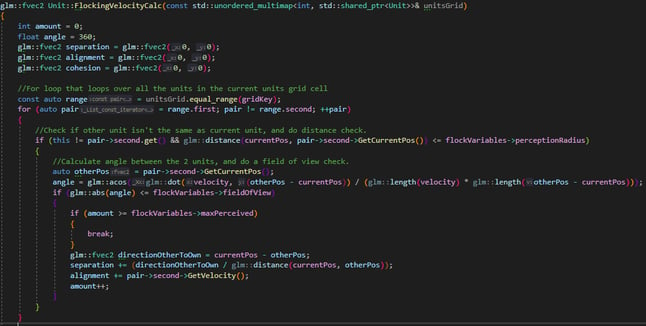
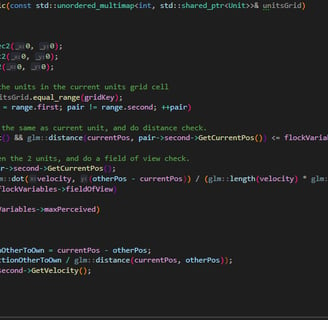
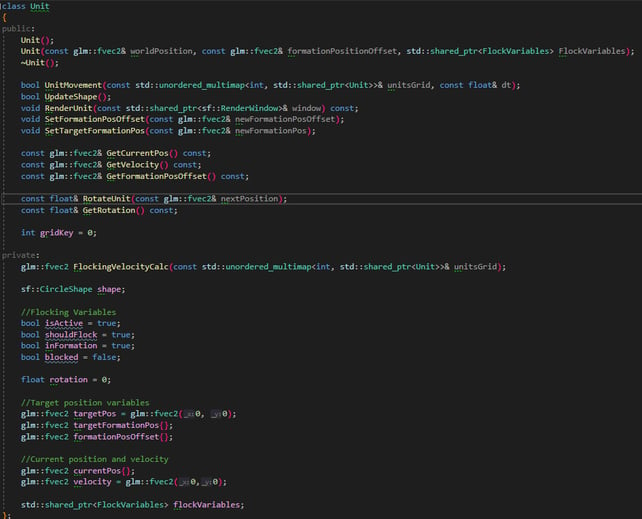
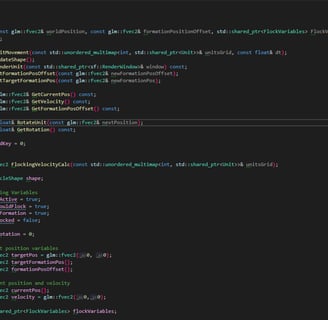